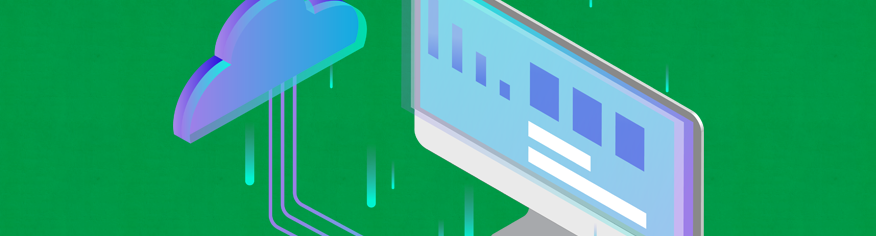
How to make a simple image uploader
A step-by-step guide on how to make a simple image uploader script in PHP. In today’s digital age, uploading images is a common requirement for many web applications. Whether you’re building a social media platform, an e-commerce website, or a blogging platform, users often need to upload images to share with others. In this context, knowing how to create an image uploader script in PHP can be a valuable skill.
In this tutorial, I will guide you through the process of creating a simple image uploader script in PHP. We will start by creating an HTML form that allows users to select and upload an image. Then, we will write PHP code to handle the uploaded image, check for errors, and save it to a target directory on the server.

We will also discuss some additional security measures you can take to ensure that your script is safe and secure from any malicious attacks. By the end of this tutorial, you will have a basic understanding of how to create an image uploader script in PHP and some best practices for securing it. So, let’s get started!
Step By Step PHP Uploader
1 – Create a new PHP file and open it in your code editor.
2 – Add an HTML form with an input field of type “file” and a submit button. Set the form’s method attribute to “post” and the enctype attribute to “multipart/form-data”. This will allow the form to upload files.
<form method="post" enctype="multipart/form-data">
<input type="file" name="image">
<button type="submit">Upload Image</button>
</form>
3 – In the PHP section of your file, check if the form was submitted using the $_SERVER[‘REQUEST_METHOD’] superglobal. This will ensure that the code inside the conditional block only runs when the form is submitted.
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
// code here
}
4 – Check if the file was uploaded without errors using the $_FILES superglobal. $_FILES[‘image’] will contain information about the uploaded file, including its name, size, and temporary location on the server. Check if $_FILES[‘image’][‘error’] is equal to 0 to make sure the file was uploaded without errors.
if (isset($_FILES['image']) && $_FILES['image']['error'] == 0) {
// code here
}
5 – Set a target directory to upload the file to. This should be a directory on your server where the uploaded files will be stored.
$target_dir = "uploads/";
6 – Get the file name and extension using basename() and pathinfo(). This will allow you to generate a unique name for the file later.
$filename = basename($_FILES['image']['name']);
$extension = strtolower(pathinfo($filename, PATHINFO_EXTENSION));
7 – Generate a unique name for the file using uniqid(). This will ensure that each uploaded file has a unique name.
$new_filename = uniqid() . '.' . $extension;
8 – Set the target path for the uploaded file using the target directory and the unique name.
$target_path = $target_dir . $new_filename;
9 – Check if the file is an image using getimagesize(). This function returns an array of information about the image, including its width, height, and type. Check if the function returns a value other than false to make sure the uploaded file is an image.
$is_image = getimagesize($_FILES['image']['tmp_name']);
if ($is_image !== false) {
// code here
} else {
// error message
}
10 – Upload the file to the target directory using move_uploaded_file(). This function moves the uploaded file from its temporary location on the server to the target directory.
if (move_uploaded_file($_FILES['image']['tmp_name'], $target_path)) {
// success message
} else {
// error message
}
11 – Add success and error messages to let the user know if the upload was successful or not.
if (move_uploaded_file($_FILES['image']['tmp_name'], $target_path)) {
echo "The file " . $new_filename . " has been uploaded.";
} else {
echo "Sorry, there was an error uploading your file.";
}
12 – Save the file and upload it to your server. You should now be able to upload images using the HTML form.
Note: This is a basic script that does not include any security measures or sanitization checks. It is important to add additional security measures such as file type validation, file size limitations, and proper sanitization checks to prevent any security vulnerabilities.
13 – Add file type validation to make sure the uploaded file is an image. This can be done by checking the file’s extension or MIME type. Here’s an example using the file extension:
$allowed_extensions = ['jpg', 'jpeg', 'png', 'gif'];
$extension = strtolower(pathinfo($filename, PATHINFO_EXTENSION));
if (!in_array($extension, $allowed_extensions)) {
// error message
}
14 – Add file size limitations to prevent users from uploading large files that could cause performance issues. This can be done by checking the file’s size against a maximum size limit.
$max_size = 500000; // 500KB
if ($_FILES['image']['size'] > $max_size) {
// error message
}
15 – Add proper sanitization checks to prevent any security vulnerabilities. This can be done by using functions such as htmlspecialchars() and strip_tags() to sanitize user input.
$filename = htmlspecialchars(basename($_FILES['image']['name']));
16 – Lastly, don’t forget to secure the upload directory by setting appropriate permissions and making sure it is not accessible via the web.
chmod($target_dir, 0755);
And there you have it! A basic image uploader script in PHP with some additional security measures. Remember to test your script thoroughly before deploying it to a production environment.
In conclusion, creating an image uploader script in PHP is a relatively simple process that can be useful in a variety of web applications. By following the steps outlined in this tutorial, you should now have a basic understanding of how to create a functional image uploader script in PHP. Additionally, we have discussed some important security measures to implement to ensure that your script is safe and secure. As always, it’s important to thoroughly test your script and follow best practices when deploying it to a production environment.